Step-by-Step Guide: Creating a React TypeScript App from Scratch
React, developed and maintained by Facebook (now Meta), is a powerful JavaScript library that has revolutionized the way developers build user interfaces. Its component-based architecture allows developers to create reusable UI elements, making it easier to manage complex applications and maintain clean, organized code. The virtual DOM implementation in React ensures efficient rendering and optimal performance, while its unidirectional data flow helps maintain predictable state management.
When combined with TypeScript, a statically typed superset of JavaScript, React development reaches new heights of reliability and maintainability. TypeScript adds strong typing, interfaces, and advanced object-oriented features to React applications, enabling developers to catch potential errors during development rather than at runtime. This combination also provides excellent IDE support with features like intelligent code completion, refactoring, and inline documentation.
To start building React applications with TypeScript, developers typically use one of two popular bootstrapping solutions:
- Create React App (CRA) with TypeScript template
- Next.js with TypeScript
Both solutions provide robust development environments with hot reloading, built-in testing support, and production build optimization, making it easier for developers to focus on building features rather than configuring build tools.
Let's explore in detail how to work with Create React App and Next.js.
Creating a React App with Create React App
Create React App(CRA) is a popular tool that helps developers quickly set up a new React project without dealing with complex configuration. It creates a complete React development environment with a single command, including all the necessary build tools and dependencies. CRA is officially maintained by the React team at Facebook and is considered the best way to start building a new single-page React application.
With CRA, developers can focus on writing code instead of spending time on build configuration. It includes features like hot reloading, built-in testing, and production build optimization out of the box.
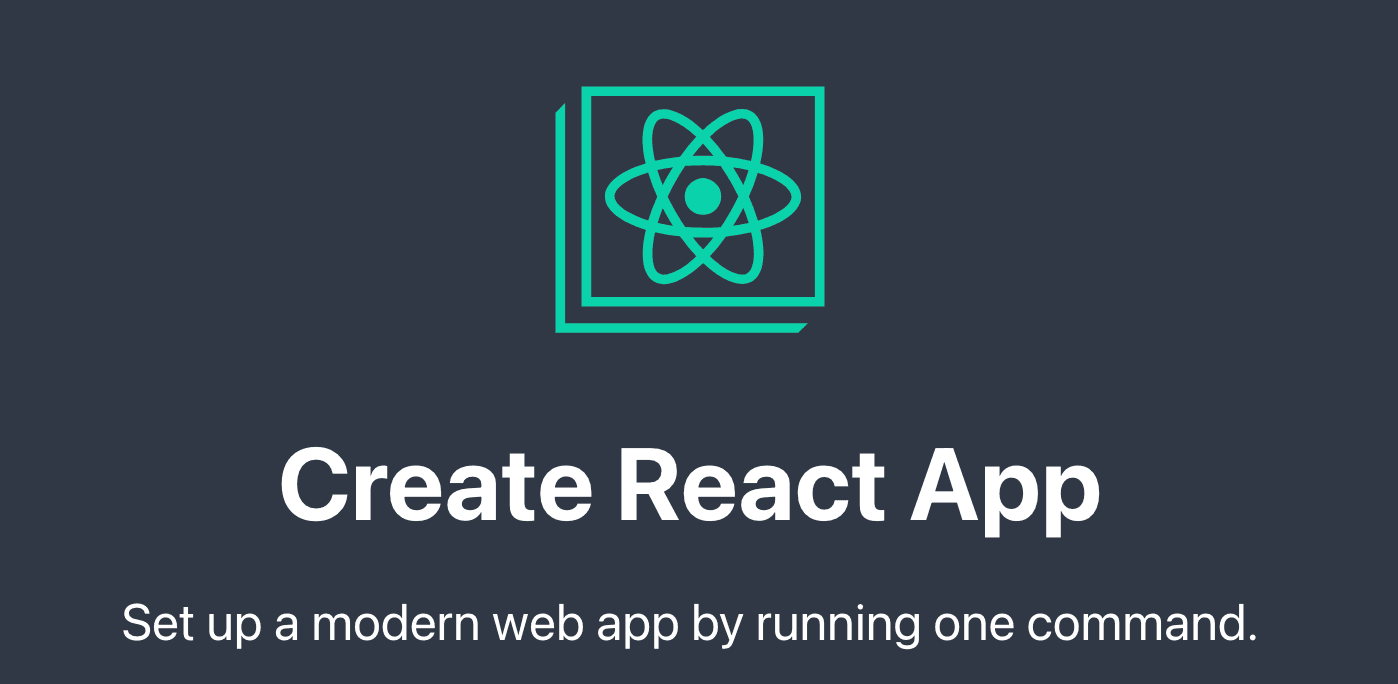
Here are the detailed steps for creating one:
Step 1: Install Node.js
Before you start, ensure that you have Node.js installed on your machine. You can download it from nodejs.org. The installation will also include npm, which is the package manager for Node.js.
Step 2: Create a New React App
You can use the create-react-app command-line tool to set up a new React application with TypeScript. Open your terminal or command prompt and run the following command:
npx create-react-app my-app --template typescript
Step 3: Navigate to Your Project Directory
Once the setup is complete, navigate into your project directory:
cd my-app
Your new React app will have a structure similar to this:
my-app/
├── node_modules/
├── public/
│ ├── index.html
│ └── ...
├── src/
│ ├── App.tsx
│ ├── index.tsx
│ └── ...
├── package.json
├── tsconfig.json
└── ...
- src/index.tsx: This is the entry point of your application.
- src/App.tsx: This is the main application component where you can start building your UI.
- tsconfig.json: The
tsconfig.json
file is where you configure TypeScript options. CRA provides a sensible default configuration, but you can customize it according to your needs.
Step 4: Start the Development Server
You can now start the development server to see your new React app in action. Run the following command:
npm start
This command will start the development server and open your new React app in your default web browser, usually at http://localhost:3000.
Step 5: Start Coding with TypeScript
You can now start writing your React components using TypeScript. Here’s a simple example of a functional component:
// src/App.tsx
import React from 'react';
const App: React.FC = () => {
return (
<div>
<h1>Hello, TypeScript with React!</h1>
</div>
);
};
export default App;
In this example, we define a functional component App using TypeScript. The React.FC type is used to indicate that this is a functional component.
Step 6: Build Your Application
Once you have developed your application and are ready to deploy it, you can build it for production:
npm run build
This command will create an optimized production build in the build directory.
Creating a React App with Next.js
Next.js is a popular React framework created by Vercel that makes it easier to build fast and modern web applications. It provides features like server-side rendering (SSR), static site generation (SSG), and automatic routing out of the box. Next.js simplifies the development process by handling many complex configurations automatically, allowing developers to focus more on building their applications rather than setting up infrastructure.
Below are the detailed steps for creating a Next.js app:
Step 1: Install Node.js
Ensure that you have Node.js installed on your machine.
Step 2: Create a New Next.js App
Use the following command to create a new Next.js app with TypeScript:
npx create-next-app@latest my-next-app --typescript
Replace my-next-app with your desired project name.
Step 3: Navigate to Your Project Directory
cd my-next-app
Step 4: Start the Development Server
npm run dev
This will start the development server, and you can view your app in the browser at http://localhost:3000.
Step 5: Project Structure
The created project structure will look something like this:
my-next-app/
├── node_modules/
├── public/ # Static files (images, fonts, etc.)
├── src/
│ ├── app/ # App router directory (Next.js 13+)
│ │ ├── layout.tsx
│ │ └── page.tsx
│ ├── components/ # Reusable React components
│ │ └── Button.tsx
│ ├── styles/ # CSS/SCSS files
│ │ └── globals.css
│ └── types/ # TypeScript type definitions
│ └── index.ts
├── .gitignore
├── next.config.js # Next.js configuration
├── package.json # Project dependencies and scripts
├── tsconfig.json # TypeScript configuration
└── README.md
Key directories and files:
- public/: Contains static assets like images and fonts
- src/: Main source code directory
- src/app/: App Router directory (Next.js 13+)
- next.config.js: Next.js configuration
- tsconfig.json: TypeScript configuration
Step 6: Adding TypeScript Types
You can start using TypeScript types in your page components. For example:
// src/app/page.tsx
import React from 'react';
const Home: React.FC = () => {
return (
<div>
<h1>Welcome to Next.js with TypeScript!</h1>
</div>
);
};
export default Home;
Step 7: Export Static Site
First, create an optimized production build of your Next.js application.
npm run build
Then, export to static HTML version of your application. It generates a standalone `out` directory with completely static assets (HTML, CSS, JavaScript files). This is useful when you want to deploy your site to static hosting platforms like EdgeOne Pages.
next export
Deploying a React App with EdgeOne Pages
EdgeOne Pages is a front-end development and deployment platform built on Tencent's EdgeOne infrastructure, specifically designed for modern web development. It empowers developers to quickly build and deploy static sites and serverless applications.
Unlike other deployment platforms, EdgeOne Pages stands out by integrating edge functions, which ensures efficient content delivery and dynamic functionality expansion. This integration provides a significant advantage in user access speed, delivering a seamless experience for users worldwide.
Below is a diagram illustrating the deployment process:
For a more detailed guide, please refer to this operation guide.
Conclusion
Congratulations on successfully building and deploying your React TypeScript application! This achievement marks an important milestone in your development journey. The combination of React and TypeScript enables you to create robust, type-safe applications with confidence. To further enhance your development workflow, consider leveraging modern hosting platforms like EdgeOne Pages, which offer seamless deployment experiences.
Ready to embark on your next project? Start deploying with just one click - absolutely free.