Signature URL
Operation scenarios
A Signature URL refers to a URL link generated by dynamically modifying image element variables based on an image template, and used for sharing. This article will introduce how to generate a Signature URL link.
Prerequisites
1. Activate Open Edge
2. Get the Image Rendering API Keys
Obtain the Security Credential required to generate the signed URL, which is the API Key, by following these steps:
After opening the image template, switch to Settings, and check the API Key item.
Note:
The API Key is used to generate signature links and for API calls. It also identifies the uniqueness of the account. Please keep your API Key secure to avoid leakage.
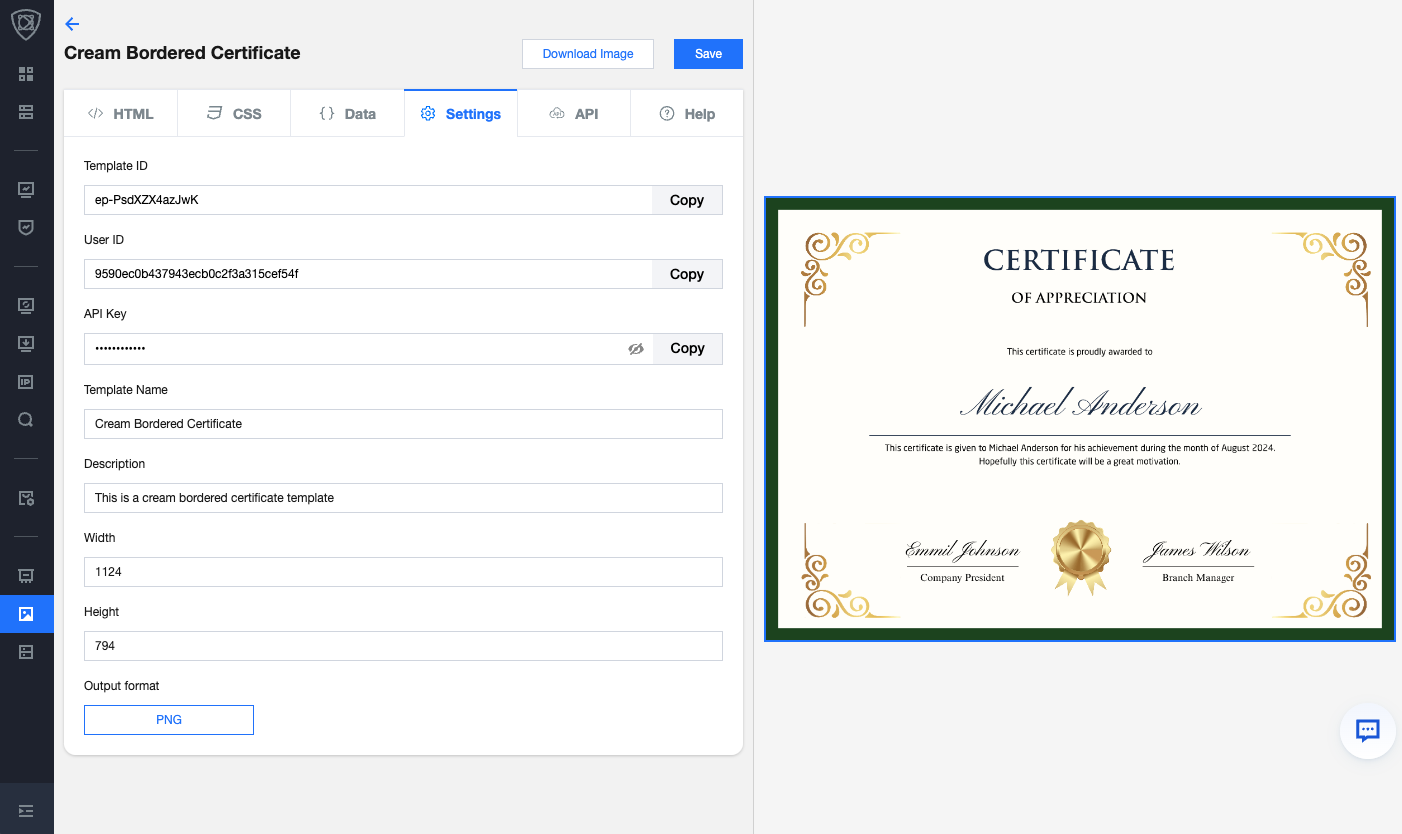
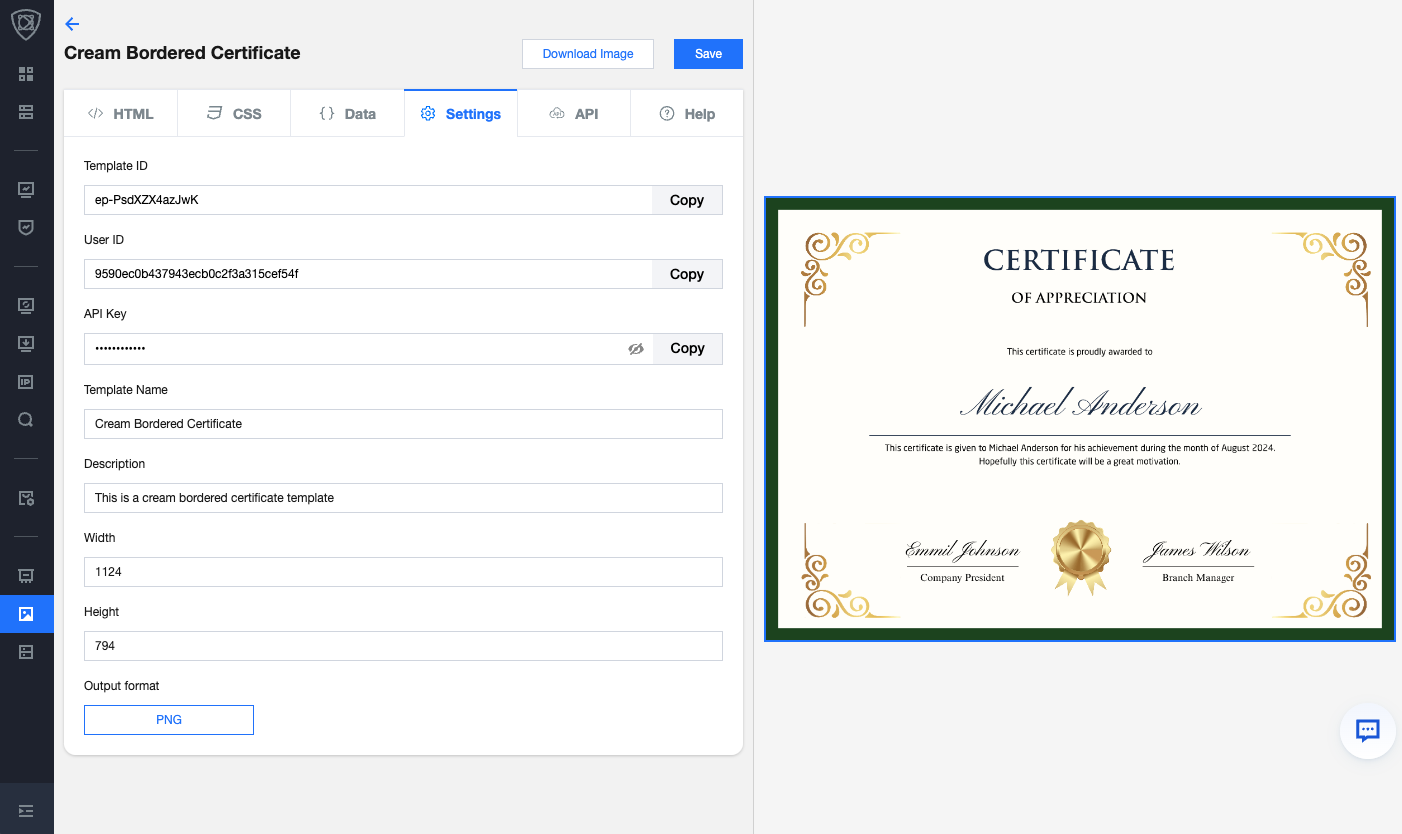
Sample code
To facilitate user development, image rendering provides demos for multiple programming languages. For details, please refer to below:
const crypto = require('crypto');function generateMD5(input) {return crypto.createHash('md5').update(input).digest('hex');}// The image format set in the template can be viewed in Settingsconst format = 'png';// User ID can be viewed in Settingsconst userId = '6b4afbd3d1834aa0a5537e5b02ddab3e';// Template ID can be viewed in Settingsconst templateId = 'ep-Zl1EQGmDexeY';// API Key used to generate signatures can be viewed in Settingsconst apiKey = '$YOUR_API_KEY$';/** Please fill in the template variable parameters to be modified here! */const params = {title: 'Hello, Edge',};// Sort the parameter keysconst sortedKeys = Object.keys(params).sort();// Concatenate the parametersconst searchParams = sortedKeys.map(key => `${key}=${params[key]}`).join('&');// Data to be signedconst signData = JSON.stringify({apiKey: apiKey,searchParams: searchParams,});// Call md5 to generate signatureconst sign = generateMD5(signData);// Execute encodeURIComponent on the values of URL parameters to encode special characters in the URLconst encodedSearchParams = sortedKeys.map(key => `${key}=${encodeURIComponent(params[key])}`).join('&');const finalUrl = `https://image.edgeone.app/${sign}/${userId}/${templateId}.${format}?${encodedSearchParams}`;console.log(finalUrl);// Sample printed URL: https://image.edgeone.app/c640ab6cb550eaf87fd13d7bb716370f/6b4afbd3d1834aa0a5537e5b02ddab3e/ep-Zl1EQGmDexeY.png
package mainimport ("crypto/md5""encoding/hex""encoding/json""fmt""net/url""sort")func generateMD5(input string) string {hash := md5.New()hash.Write([]byte(input))return hex.EncodeToString(hash.Sum(nil))}func main() {// The image format set in the template can be viewed in Settingsformat := "png"// User ID can be viewed in SettingsuserId := "6b4afbd3d1834aa0a5537e5b02ddab3e"// Template ID can be viewed in SettingstemplateId := "ep-Zl1EQGmDexeY"// API Key used to generate signatures can be viewed in SettingsapiKey := "$YOUR_API_KEY$"/** Please fill in the template variable parameters to be modified here! */params := map[string]string{"title": "Hello, Edge",}// Sort the parameter keyssortedKeys := make([]string, 0, len(params))for key := range params {sortedKeys = append(sortedKeys, key)}sort.Strings(sortedKeys)// Concatenate the parameterssearchParams := ""for i, key := range sortedKeys {if i > 0 {searchParams += "&"}searchParams += fmt.Sprintf("%s=%s", key, params[key])}// Data to be signedsignData := map[string]interface{}{"apiKey": apiKey,"searchParams": searchParams,}signDataJSON, _ := json.Marshal(signData)// Call md5 to generate signaturesign := generateMD5(string(signDataJSON))// Execute encodeURIComponent on the values of URL parameters to encode special characters in the URLencodedSearchParams := ""for i, key := range sortedKeys {if i > 0 {encodedSearchParams += "&"}encodedValue := url.QueryEscape(params[key])encodedSearchParams += fmt.Sprintf("%s=%s", key, encodedValue)}finalUrl := fmt.Sprintf("https://image.edgeone.app/%s/%s/%s.%s?%s", sign, userId, templateId, format, encodedSearchParams)fmt.Println(finalUrl)// Sample printed URL: https://image.edgeone.app/c640ab6cb550eaf87fd13d7bb716370f/6b4afbd3d1834aa0a5537e5b02ddab3e/ep-Zl1EQGmDexeY.png}
import java.security.MessageDigest;import java.security.NoSuchAlgorithmException;import java.net.URLEncoder;import java.nio.charset.StandardCharsets;import java.util.HashMap;import java.util.Map;import java.util.TreeMap;public class Main {// Generate MD5 hashpublic static String generateMD5(String input) {try {MessageDigest md = MessageDigest.getInstance("MD5");byte[] messageDigest = md.digest(input.getBytes(StandardCharsets.UTF_8));StringBuilder hexString = new StringBuilder();for (byte b : messageDigest) {String hex = Integer.toHexString(0xff & b);if (hex.length() == 1) hexString.append('0');hexString.append(hex);}return hexString.toString();} catch (NoSuchAlgorithmException e) {throw new RuntimeException(e);}}public static void main(String[] args) {// The image format set in the template can be viewed in SettingsString format = "png";// User ID can be viewed in SettingsString userId = "6b4afbd3d1834aa0a5537e5b02ddab3e";// Template ID can be viewed in SettingsString templateId = "ep-Zl1EQGmDexeY";// API Key used to generate signatures can be viewed in SettingsString apiKey = "$YOUR_API_KEY$";Map<String, String> params = new HashMap<>();/** Please fill in the template variable parameters to be modified here! */params.put("title", "Hello, Edge");// Sort the parameter keysTreeMap<String, String> sortedParams = new TreeMap<>(params);// Concatenate the parametersStringBuilder searchParams = new StringBuilder();for (Map.Entry<String, String> entry : sortedParams.entrySet()) {if (searchParams.length() > 0) {searchParams.append("&");}String escapedValue = entry.getValue().replace("\"", "\\\"");searchParams.append(entry.getKey()).append("=").append(escapedValue);}// Data to be signedString signData = String.format("{\"apiKey\":\"%s\",\"searchParams\":\"%s\"}", apiKey, searchParams.toString());// Call md5 to generate signatureString sign = generateMD5(signData);// Execute encodeURIComponent on the values of URL parameters to encode special characters in the URLStringBuilder encodedSearchParams = new StringBuilder();for (Map.Entry<String, String> entry : sortedParams.entrySet()) {if (encodedSearchParams.length() > 0) {encodedSearchParams.append("&");}String encodedValue = URLEncoder.encode(entry.getValue(), StandardCharsets.UTF_8);encodedSearchParams.append(entry.getKey()).append("=").append(encodedValue);}String finalUrl = String.format("https://image.edgeone.app/%s/%s/%s.%s?%s", sign, userId, templateId, format, encodedSearchParams.toString());System.out.println(finalUrl);// Sample printed URL: https://image.edgeone.app/c640ab6cb550eaf87fd13d7bb716370f/6b4afbd3d1834aa0a5537e5b02ddab3e/ep-Zl1EQGmDexeY.png}}
<?php// Generate MD5 hashfunction generateMD5($input) {return md5($input);}// The image format set in the template can be viewed in Settings$format = 'png';// User ID can be viewed in Settings$userId = '6b4afbd3d1834aa0a5537e5b02ddab3e';// Template ID can be viewed in Settings$templateId = 'ep-Zl1EQGmDexeY';// API Key used to generate signatures can be viewed in Settings$apiKey = '$YOUR_API_KEY$';/** Please fill in the template variable parameters to be modified here! */$params = ['title' => 'Hello, Edge',];// Sort the parameter keysksort($params);// Concatenate the parameters$searchParams = '';foreach ($params as $key => $value) {if ($searchParams !== '') {$searchParams .= '&';}$searchParams .= $key . '=' . $value;}// Data to be signed$signData = json_encode(['apiKey' => $apiKey,'searchParams' => $searchParams,]);// Call md5 to generate signature$sign = generateMD5($signData);// Execute urlencode on the values of URL parameters to encode special characters in the URL$encodedSearchParams = http_build_query($params);// Build the final URL$finalUrl = sprintf('https://image.edgeone.app/%s/%s/%s.%s?%s',$sign,$userId,$templateId,$format,$encodedSearchParams);echo $finalUrl;// Sample printed URL: https://image.edgeone.app/c640ab6cb550eaf87fd13d7bb716370f/6b4afbd3d1834aa0a5537e5b02ddab3e/ep-Zl1EQGmDexeY.png?>
import hashlibimport urllib.parse# Generate MD5 hashdef generate_md5(input_string):return hashlib.md5(input_string.encode('utf-8')).hexdigest()# The image format set in the template can be viewed in Settingsformat = 'png'# User ID can be viewed in Settingsuser_id = '6b4afbd3d1834aa0a5537e5b02ddab3e'# Template ID can be viewed in Settingstemplate_id = 'ep-Zl1EQGmDexeY'# API Key used to generate signatures can be viewed in Settingsapi_key = '$YOUR_API_KEY$'# Please fill in the template variable parameters to be modified here!params = {'title': 'Hello, Edge',}# Sort the parameter keyssorted_params = sorted(params.items())# Concatenate the parameters without URL encoding the valuessearch_params = '&'.join(f"{key}={value.replace('\"', '\\\"')}" for key, value in sorted_params)# Data to be signedsign_data = f'{{"apiKey":"{api_key}","searchParams":"{search_params}"}}'print(sign_data)# Call md5 to generate signaturesign = generate_md5(sign_data)# URL encode the values of the URL parametersencoded_search_params = '&'.join(f"{key}={urllib.parse.quote(value)}" for key, value in sorted_params)# Build the final URLfinal_url = f'https://image.edgeone.app/{sign}/{user_id}/{template_id}.{format}?{encoded_search_params}'print(final_url)# Sample printed URL: https://image.edgeone.app/c640ab6cb550eaf87fd13d7bb716370f/6b4afbd3d1834aa0a5537e5b02ddab3e/ep-Zl1EQGmDexeY.png