API Generation
Operation scenarios
API generation refers to dynamically modifying image element variables based on an image template and then invoking the API to generate image data. This article will introduce how to call the API.
Prerequisites
1. Activate Open Edge
2. Get the Image Renderer API Keys
To obtain the security credentials required to invoke the API, i.e., API key, follow these steps:
After opening the image template, switch to Settings, and check the API Key item.
Note:
The API key is used to generate encrypted links and API calls, and it is used to identify the uniqueness of the account. Please keep your API key secure to prevent leakage.
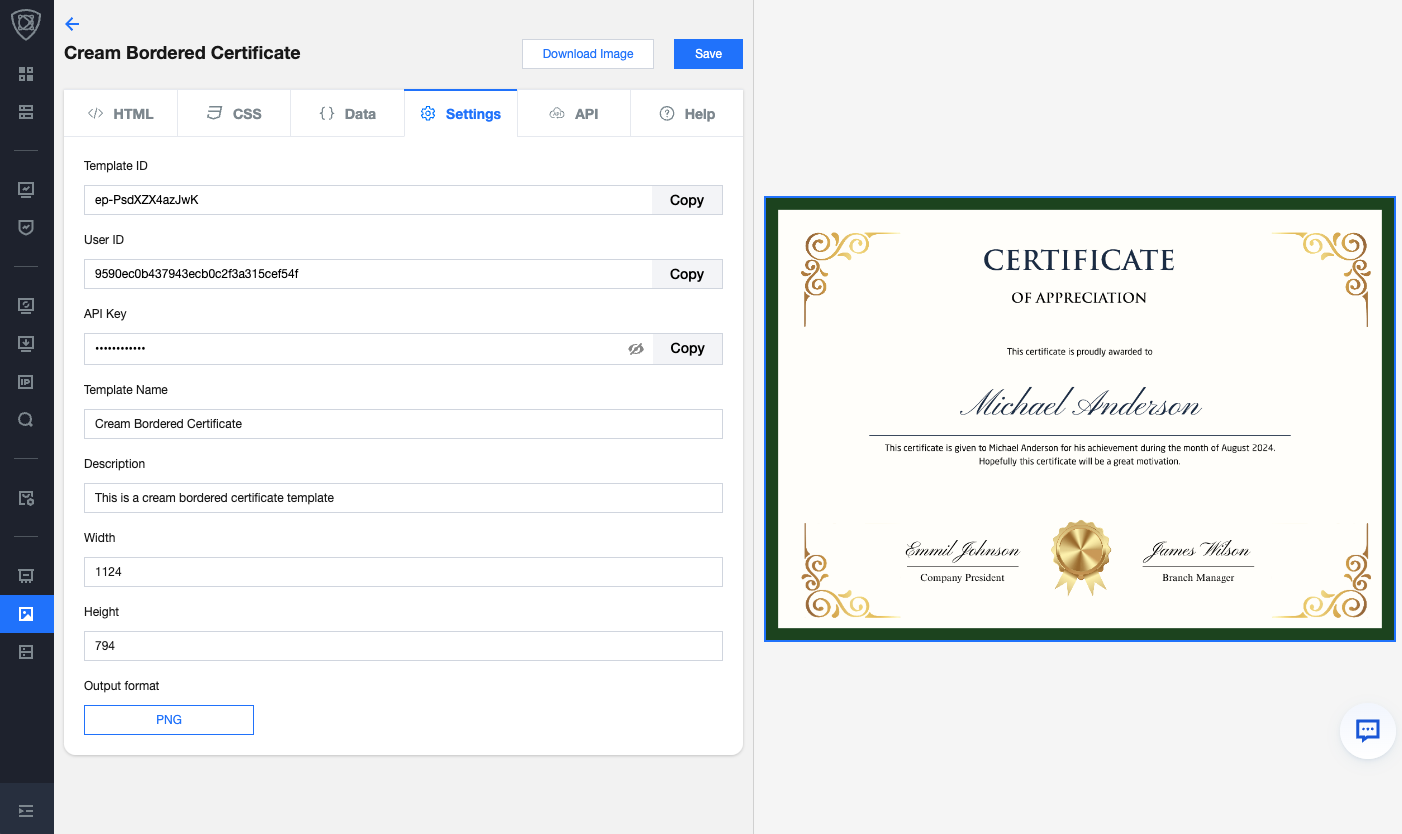
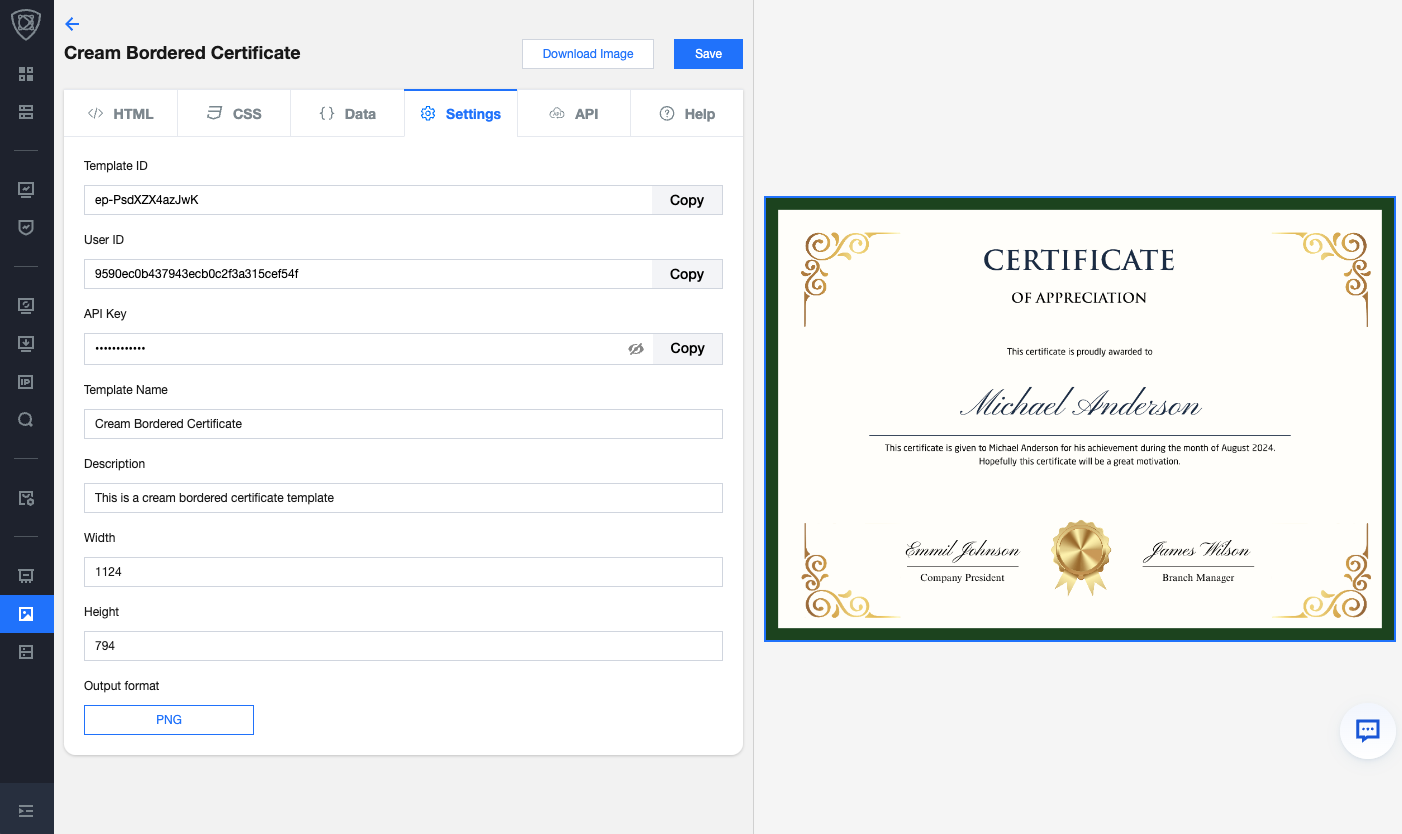
Sample code
For user convenience, the following code demonstrates calling the API to generate image data and saving the image data as an image. Image rendering provides multi-language demos. For details, please see below:
const axios = require('axios');const fs = require('fs');const url = 'https://image.edgeone.app/';const headers = {'Content-Type': 'application/json','OE-USER-ID': '$YOUR_USER_ID$', // User ID, can be viewed in Settings'OE-API-KEY': '$YOUR_API_KEY$', // API Key, can be viewed in Settings'OE-TEMPLATE-ID': '$TEMPLATE_ID$' // Template ID, can be viewed in Settings};const data = {title: "Hello, Edge"};axios.post(url, data, { headers, responseType: 'arraybuffer' }).then(response => {// The response is binary data, saved as an image file for demonstration purposesfs.writeFileSync('image.png', response.data);console.log('[Success]Image saved as image.png');}).catch(error => {console.error('[Error]fetching the image:', error);});
package mainimport ("bytes""encoding/json""fmt""io/ioutil""net/http""os")func main() {url := "https://image.edgeone.app/"headers := map[string]string{"Content-Type": "application/json","OE-USER-ID": "$YOUR_USER_ID$", // User ID, can be viewed in Settings"OE-API-KEY": "$YOUR_API_KEY$", // API Key, can be viewed in Settings"OE-TEMPLATE-ID": "$TEMPLATE_ID$", // Template ID, can be viewed in Settings}data := map[string]string{"title": "Hello, Edge",}// Encode data to JSONjsonData, err := json.Marshal(data)if err != nil {fmt.Println("[Error]encoding data to JSON:", err)return}// Create an HTTP requestreq, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonData))if err != nil {fmt.Println("[Error]creating HTTP request:", err)return}// Set request headersfor key, value := range headers {req.Header.Set(key, value)}// Send the requestclient := &http.Client{}resp, err := client.Do(req)if err != nil {fmt.Println("[Error]fetching the image:", err)return}defer resp.Body.Close()// Read response databody, err := ioutil.ReadAll(resp.Body)if err != nil {fmt.Println("[Error]reading response body:", err)return}// Save as an image fileerr = ioutil.WriteFile("image.png", body, 0644)if err != nil {fmt.Println("[Error]saving image:", err)return}fmt.Println("[Success]Image saved as image.png")}
import java.io.BufferedOutputStream;import java.io.FileOutputStream;import java.io.IOException;import java.io.InputStream;import java.net.HttpURLConnection;import java.net.URL;import java.nio.charset.StandardCharsets;import java.util.HashMap;import java.util.Map;import com.google.gson.Gson;public class Main {public static void main(String[] args) {String urlString = "https://image.edgeone.app/";Map<String, String> headers = new HashMap<>();headers.put("Content-Type", "application/json");headers.put("OE-USER-ID", "$YOUR_USER_ID$"); // User ID, can be viewed in Settingsheaders.put("OE-API-KEY", "$YOUR_API_KEY$"); // API Key, can be viewed in Settingsheaders.put("OE-TEMPLATE-ID", "$TEMPLATE_ID$"); // Template ID, can be viewed in SettingsMap<String, String> data = new HashMap<>();data.put("title", "Hello, Edge");Gson gson = new Gson();String jsonData = gson.toJson(data);try {URL url = new URL(urlString);HttpURLConnection connection = (HttpURLConnection) url.openConnection();connection.setRequestMethod("POST");connection.setDoOutput(true);// Set request headersfor (Map.Entry<String, String> entry : headers.entrySet()) {connection.setRequestProperty(entry.getKey(), entry.getValue());}// Send the request bodytry (BufferedOutputStream out = new BufferedOutputStream(connection.getOutputStream())) {out.write(jsonData.getBytes(StandardCharsets.UTF_8));}// Get the responseint responseCode = connection.getResponseCode();if (responseCode == HttpURLConnection.HTTP_OK) {try (InputStream in = connection.getInputStream();FileOutputStream fileOutputStream = new FileOutputStream("image.png")) {byte[] buffer = new byte[1024];int bytesRead;while ((bytesRead = in.read(buffer)) != -1) {fileOutputStream.write(buffer, 0, bytesRead);}System.out.println("[Success]Image saved as image.png");}} else {System.out.println("[Error]fetching the image: HTTP error code " + responseCode);}} catch (IOException e) {System.err.println("[Error]fetching the image: " + e.getMessage());}}}
<?php$url = 'https://image.edgeone.app/';$headers = ['Content-Type: application/json','OE-USER-ID: $YOUR_USER_ID$', // User ID, can be viewed in Settings'OE-API-KEY: $YOUR_API_KEY$', // API Key, can be viewed in Settings'OE-TEMPLATE-ID: $TEMPLATE_ID$' // Template ID, can be viewed in Settings];$data = ['title' => 'Hello, Edge'];// Encode data as JSON$jsonData = json_encode($data);// Initialize cURL session$ch = curl_init($url);// Set cURL optionscurl_setopt($ch, CURLOPT_POST, 1);curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);curl_setopt($ch, CURLOPT_BINARYTRANSFER, true);// Execute cURL request$response = curl_exec($ch);// Check for errorsif (curl_errno($ch)) {echo '[Error]fetching the image: ' . curl_error($ch);} else {// Save image filefile_put_contents('image.png', $response);echo '[Success]Image saved as image.png';}// Close cURL sessioncurl_close($ch);?>
import requestsimport jsonurl = 'https://image.edgeone.app/'headers = {'Content-Type': 'application/json','OE-USER-ID': '$YOUR_USER_ID$', # User ID, can be viewed in Settings'OE-API-KEY': '$YOUR_API_KEY$', # API Key, can be viewed in Settings'OE-TEMPLATE-ID': '$TEMPLATE_ID$' # Template ID, can be viewed in Settings}data = {'title': 'Hello, Edge'}# Encode data as JSONjson_data = json.dumps(data)try:# Send POST requestresponse = requests.post(url, headers=headers, data=json_data, stream=True)# Check if the request is successfulresponse.raise_for_status()# Save image filewith open('image.png', 'wb') as file:for chunk in response.iter_content(chunk_size=8192):file.write(chunk)print('[Success]Image saved as image.png')except requests.exceptions.RequestException as e:print(f'[Error]fetching the image: {e}')