API 生成
操作场景
API 生成是指基于图片模板动态修改图片元素变量后调用API生成图片数据,本文将介绍如何调用 API。
前提条件
1. 开通 Open Edge
2. 获取图片渲染 API 密钥
获取调用 API 所需的安全凭证,即 API Key,步骤如下:
打开图片模板后,切换到 Settings,查看 API Key 一项。
注意:
API Key 用于生成加密链接及 API 调用,并被用来识别账号的唯一性,请保管好您的 API Key 避免泄漏。
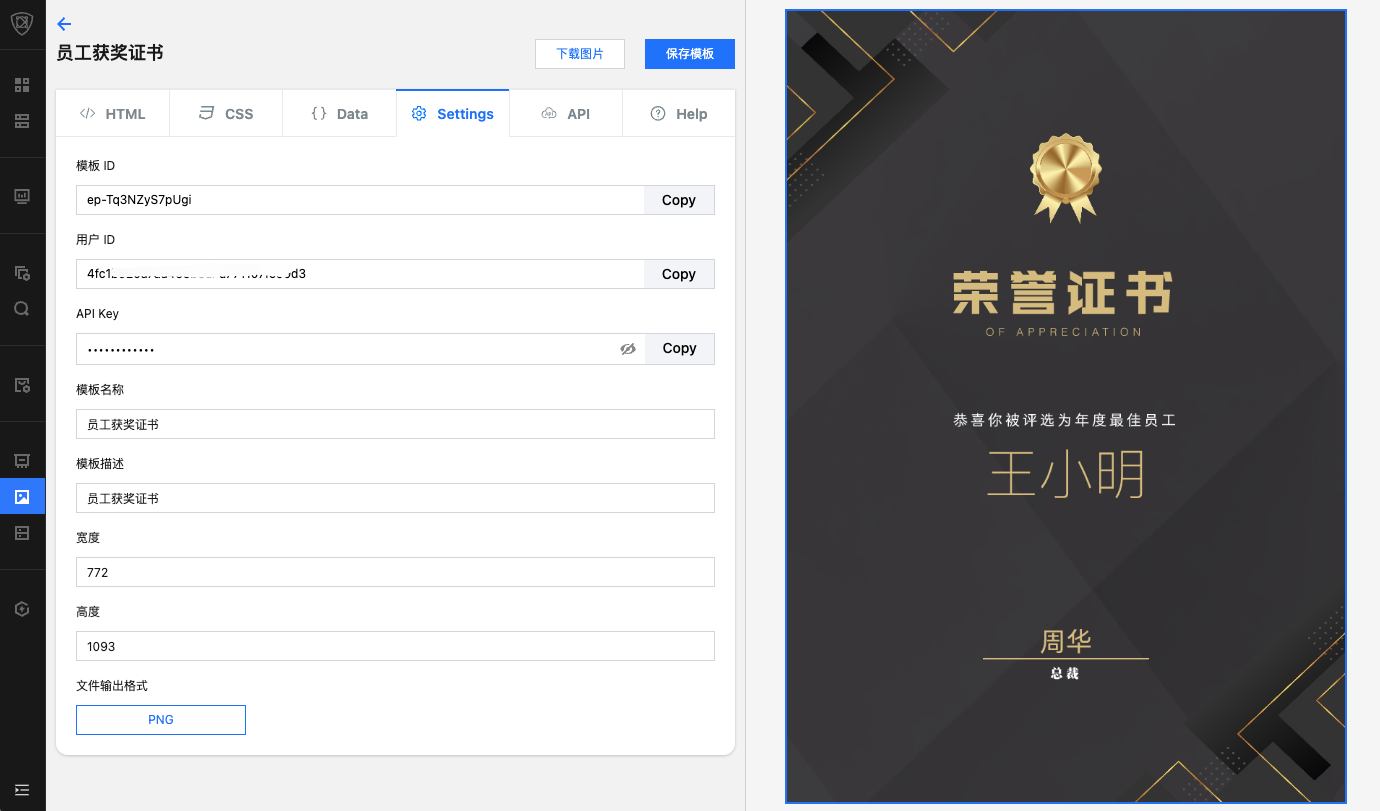
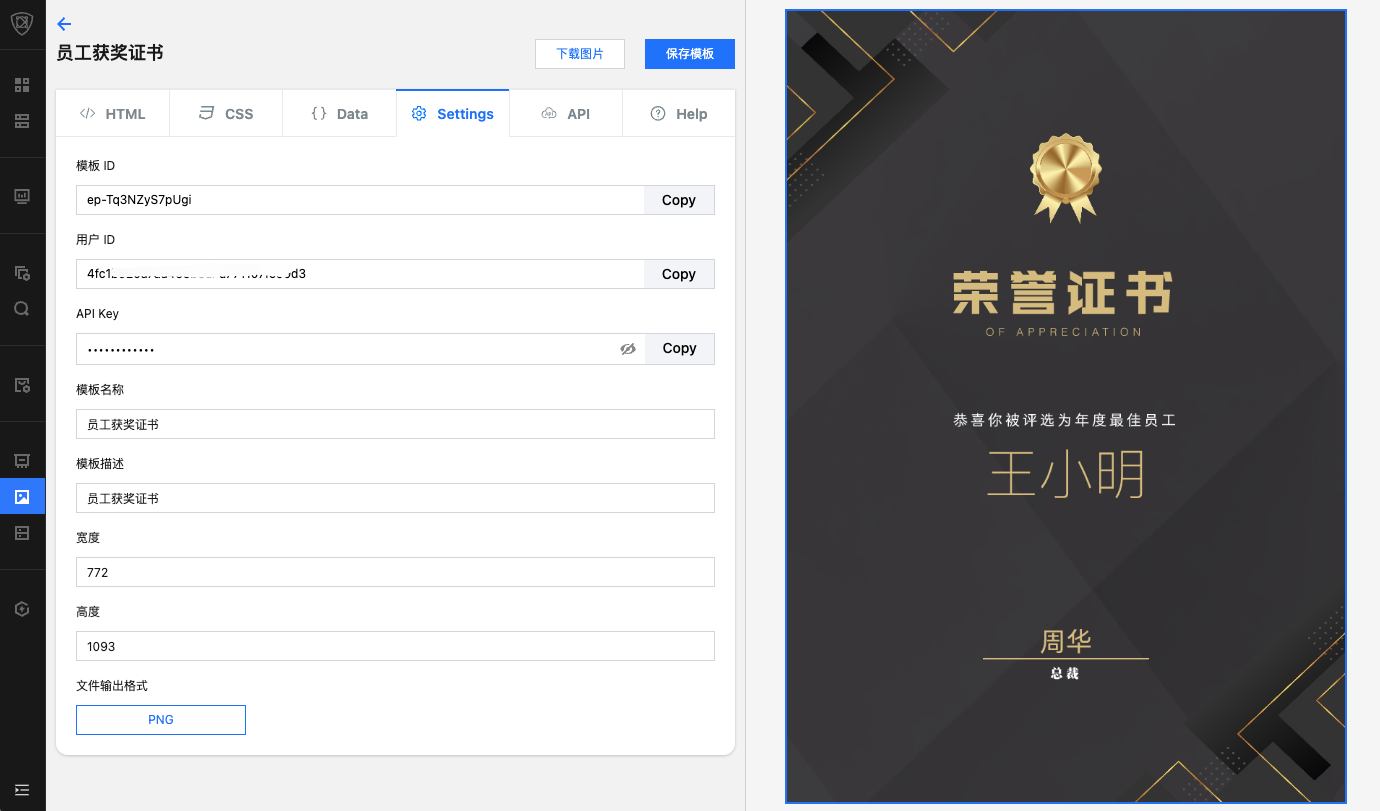
示例代码
为了方便用户开发,下面将代码演示通过API调用生成图片数据,将图片数据保存为图片。图片渲染提供多语言 Demo,详情请参见下方:
const axios = require('axios');const fs = require('fs');const url = 'https://image.edgeone.app/';const headers = {'Content-Type': 'application/json','OE-USER-ID': '$YOUR_USER_ID$', // 用户 Id,可在 Settings 中查看'OE-API-KEY': '$YOUR_API_KEY$', // API Key,可在 Settings 中查看'OE-TEMPLATE-ID': '$TEMPLATE_ID$' // 模板 Id,可在 Settings 中查看};const data = {title: "Hello, Edge"};axios.post(url, data, { headers, responseType: 'arraybuffer' }).then(response => {// 返回为二进制数据,为了方便演示保存为图片文件fs.writeFileSync('image.png', response.data);console.log('[Success]Image saved as image.png');}).catch(error => {console.error('[Error]fetching the image:', error);});
package mainimport ("bytes""encoding/json""fmt""io/ioutil""net/http""os")func main() {url := "https://image.edgeone.app/"headers := map[string]string{"Content-Type": "application/json","OE-USER-ID": "$YOUR_USER_ID$", // 用户 Id,可在 Settings 中查看"OE-API-KEY": "$YOUR_API_KEY$", // API Key,可在 Settings 中查看"OE-TEMPLATE-ID": "$TEMPLATE_ID$", // 模板 Id,可在 Settings 中查看}data := map[string]string{"title": "Hello, Edge",}// 将数据编码为JSONjsonData, err := json.Marshal(data)if err != nil {fmt.Println("[Error]encoding data to JSON:", err)return}// 创建HTTP请求req, err := http.NewRequest("POST", url, bytes.NewBuffer(jsonData))if err != nil {fmt.Println("[Error]creating HTTP request:", err)return}// 设置请求头for key, value := range headers {req.Header.Set(key, value)}// 发送请求client := &http.Client{}resp, err := client.Do(req)if err != nil {fmt.Println("[Error]fetching the image:", err)return}defer resp.Body.Close()// 读取响应数据body, err := ioutil.ReadAll(resp.Body)if err != nil {fmt.Println("[Error]reading response body:", err)return}// 保存为图片文件err = ioutil.WriteFile("image.png", body, 0644)if err != nil {fmt.Println("[Error]saving image:", err)return}fmt.Println("[Success]Image saved as image.png")}
import java.io.BufferedOutputStream;import java.io.FileOutputStream;import java.io.IOException;import java.io.InputStream;import java.net.HttpURLConnection;import java.net.URL;import java.nio.charset.StandardCharsets;import java.util.HashMap;import java.util.Map;import com.google.gson.Gson;public class Main {public static void main(String[] args) {String urlString = "https://image.edgeone.app/";Map<String, String> headers = new HashMap<>();headers.put("Content-Type", "application/json");headers.put("OE-USER-ID", "$YOUR_USER_ID$"); // 用户 Id,可在 Settings 中查看headers.put("OE-API-KEY", "$YOUR_API_KEY$"); // API Key,可在 Settings 中查看headers.put("OE-TEMPLATE-ID", "$TEMPLATE_ID$"); // 模板 Id,可在 Settings 中查看Map<String, String> data = new HashMap<>();data.put("title", "Hello, Edge");Gson gson = new Gson();String jsonData = gson.toJson(data);try {URL url = new URL(urlString);HttpURLConnection connection = (HttpURLConnection) url.openConnection();connection.setRequestMethod("POST");connection.setDoOutput(true);// 设置请求头for (Map.Entry<String, String> entry : headers.entrySet()) {connection.setRequestProperty(entry.getKey(), entry.getValue());}// 发送请求体try (BufferedOutputStream out = new BufferedOutputStream(connection.getOutputStream())) {out.write(jsonData.getBytes(StandardCharsets.UTF_8));}// 获取响应int responseCode = connection.getResponseCode();if (responseCode == HttpURLConnection.HTTP_OK) {try (InputStream in = connection.getInputStream();FileOutputStream fileOutputStream = new FileOutputStream("image.png")) {byte[] buffer = new byte[1024];int bytesRead;while ((bytesRead = in.read(buffer)) != -1) {fileOutputStream.write(buffer, 0, bytesRead);}System.out.println("[Success]Image saved as image.png");}} else {System.out.println("[Error]fetching the image: HTTP error code " + responseCode);}} catch (IOException e) {System.err.println("[Error]fetching the image: " + e.getMessage());}}}
<?php$url = 'https://image.edgeone.app/';$headers = ['Content-Type: application/json','OE-USER-ID: $YOUR_USER_ID$', // 用户 Id,可在 Settings 中查看'OE-API-KEY: $YOUR_API_KEY$', // API Key,可在 Settings 中查看'OE-TEMPLATE-ID: $TEMPLATE_ID$' // 模板 Id,可在 Settings 中查看];$data = ['title' => 'Hello, Edge'];// 将数据编码为JSON$jsonData = json_encode($data);// 初始化curl会话$ch = curl_init($url);// 设置curl选项curl_setopt($ch, CURLOPT_POST, 1);curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);curl_setopt($ch, CURLOPT_BINARYTRANSFER, true);// 执行curl请求$response = curl_exec($ch);// 检查是否有错误if (curl_errno($ch)) {echo '[Error]fetching the image: ' . curl_error($ch);} else {// 保存图片文件file_put_contents('image.png', $response);echo '[Success]Image saved as image.png';}// 关闭curl会话curl_close($ch);?>
import requestsimport jsonurl = 'https://image.edgeone.app/'headers = {'Content-Type': 'application/json','OE-USER-ID': '$YOUR_USER_ID$', # 用户 Id,可在 Settings 中查看'OE-API-KEY': '$YOUR_API_KEY$', # API Key,可在 Settings 中查看'OE-TEMPLATE-ID': '$TEMPLATE_ID$' # 模板 Id,可在 Settings 中查看}data = {'title': 'Hello, Edge'}# 将数据编码为JSONjson_data = json.dumps(data)try:# 发送POST请求response = requests.post(url, headers=headers, data=json_data, stream=True)# 检查请求是否成功response.raise_for_status()# 保存图片文件with open('image.png', 'wb') as file:for chunk in response.iter_content(chunk_size=8192):file.write(chunk)print('[Success]Image saved as image.png')except requests.exceptions.RequestException as e:print(f'[Error]fetching the image: {e}')